Motion Media Progress Report 2
Learn about my progress with Motion Media. I added a black coloured layer and dynamic display based on frontal face detection.
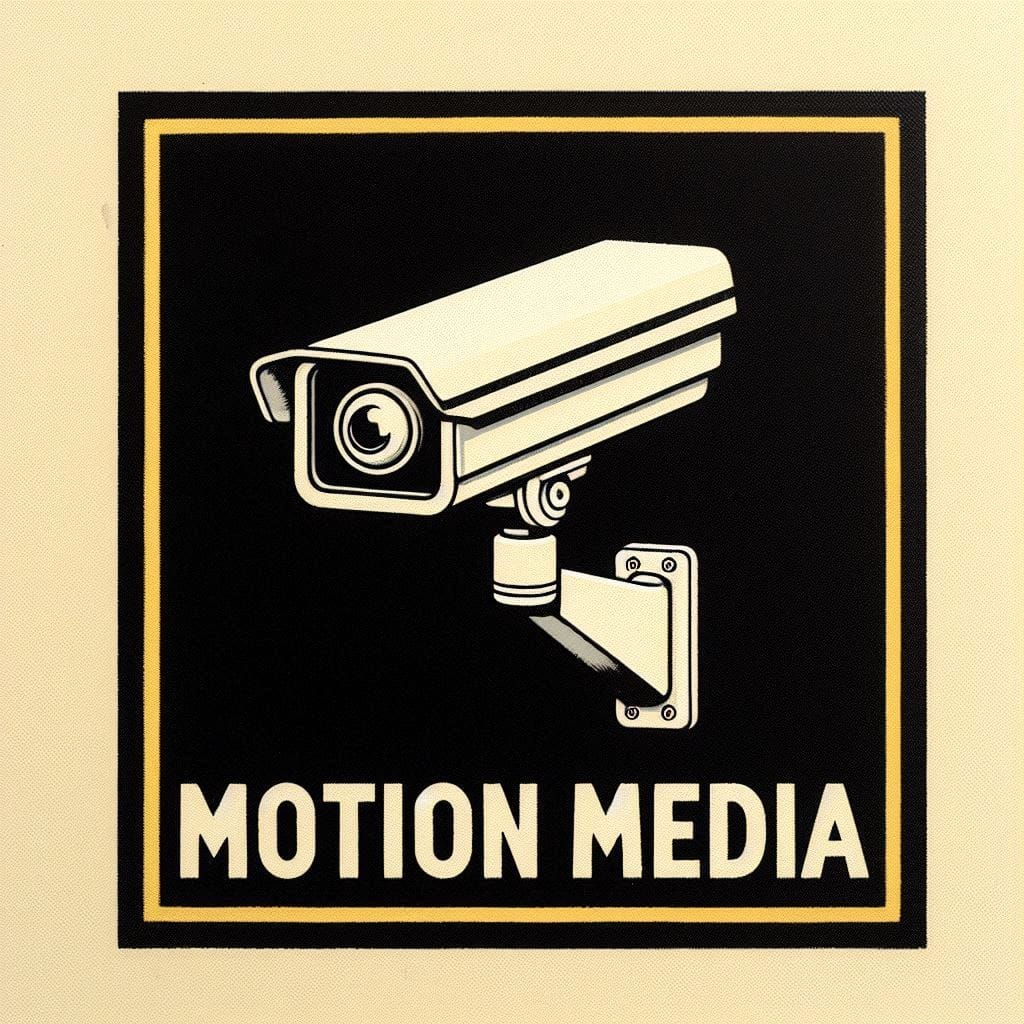
I started using the front face cascade classifier that is being provided by OpenCV because I wanted any detection to be based on whether the people being detected are looking towards the camera or not.
The ReturnDetectionInformation
is a method that belongs to the Detector class and returns a vector with information.
std::vector<cv::Rect> Detector::ReturnDetectionInformation(cv::Mat frame)
{
std::vector<cv::Rect> faces;
visionClassifier.detectMultiScale(frame, faces, 1.1, 9);
return faces;
}
The vector is being used to check if the program has found anything. It is also being used to generate bounding boxes for testing purposes.
std::vector<cv::Rect> faces = detector.ReturnDetectionInformation(frame);
for (int i = 0; i < faces.size(); i++)
{
cv::rectangle(frame, faces[i].tl(), faces[i].br(), cv::Scalar(255, 0, 255), 5);
}
At the moment the program shows or hides a video clip by adding or removing a black coloured layer. The cv::addWeighter
function is used to add the layer. The transitions between states have been smoothed out by manipulating the alpha
and beta
variables.
if (faces.empty())
{
noDetection = true;
}else
{
noDetection = false;
}
if (noDetection && alpha > 0)
{
beta += 0.1;
alpha -= 0.1;
}
if (!noDetection && alpha < 1)
{
beta -= 0.5;
alpha += 0.5;
}
cv::Mat dest;
cv::addWeighted(videoplayer.videoFrame, alpha, cv::Scalar(0, 0, 0), beta, 0.0, dest);
The result is being displayed on a full-screen window.
cv::namedWindow("Result", cv::WINDOW_NORMAL);
cv::imshow("Result", dest);
cv::setWindowProperty("Result", cv::WND_PROP_FULLSCREEN, cv::WINDOW_FULLSCREEN);
The image does not fill the window properly. This might be caused by the transition from windowed to full-screen mode. I need to work on that next.